In this tutorial I will show you how to create a New Asset Type for the Unreal Engine Editor. Unreal Engine provides a large number of Asset Types, for instance, StaticMeshes, Materials, SkeletalMeshes…
We are going to create a new type of Asset “StaticMeshVariation” that will contain a set of StaticMeshes that are variation of the same type. In our example we will use it to store all the meshes of the same type of imported mesh like doors, building stories, floors, walls…etc. so it will be easier for organize our static mesh assets.
For doing so, click on “File->New C++ Class” and it will open a new window, click on “Show all classes” and select “Object” press Next and name it “StaticMeshVariation”. Don’t modify StaticMeshVarition.cpp and Copy the following code to StaticMeshVariation.h:
STATICMESHVARIATION.H
// Created by Bionic Ape. All Rights Reserved.
#pragma once
#include "CoreMinimal.h"
#include "UObject/NoExportTypes.h"
#include "StaticMeshVariation.generated.h"
class UStaticMesh;
/**
* A set of StaticMeshes that are variation of the same type
*/
UCLASS()
class PREFABBUILDER_API UStaticMeshVariation : public UObject
{
GENERATED_BODY()
UPROPERTY(Category="PrefabBuilder", EditDefaultsOnly)
TArray<UStaticMesh*> Meshes;
};
STATICMESHVARIATION.CPP
// Created by Bionic Ape. All Rights Reserved.
#include "StaticMeshVariation.h"
Now we need a factory. Click on “File->New C++ Class”, click on “Show all classes” and select “Factory” press Next and name it “StaticMeshVariationFactory”. Copy and Paste the following code:
STATICMESHVARIATIONFACTORY.H
// Created by Bionic Ape. All Rights Reserved.
#pragma once
#include "CoreMinimal.h"
#include "Factories/Factory.h"
#include "StaticMeshVariationFactory.generated.h"
/**
*
*/
UCLASS()
class PREFABBUILDER_API UStaticMeshVariationFactory : public UFactory
{
GENERATED_BODY()
UStaticMeshVariationFactory(const FObjectInitializer& ObjectInitializer);
// UFactory interface
virtual UObject* FactoryCreateNew(UClass* Class, UObject* InParent, FName Name, EObjectFlags Flags, UObject* Context, FFeedbackContext* Warn) override;
virtual bool CanCreateNew() const override { return true; }
// End of UFactory interface
};
STATICMESHVARIATIONFACTORY.CPP
// Created by Bionic Ape. All Rights Reserved.
#include "StaticMeshVariationFactory.h"
#include "StaticMeshVariationFactory.h"
#include "StaticMeshVariation.h"
UStaticMeshVariationFactory::UStaticMeshVariationFactory(const FObjectInitializer& ObjectInitializer) : Super(ObjectInitializer) {
SupportedClass = UStaticMeshVariation::StaticClass();
bCreateNew = true;
bEditAfterNew = true;
}
UObject* UStaticMeshVariationFactory::FactoryCreateNew(UClass* Class, UObject* InParent, FName Name, EObjectFlags Flags, UObject* Context, FFeedbackContext* Warn) {
UStaticMeshVariation* NewAsset = NewObject<UStaticMeshVariation>(InParent, Class, Name, Flags);
return NewAsset;
}
Now we can go to the editor and create as many instances as we want for doing so, right click on an empty space inside the Content Browser and under “Miscelaneous” find “Static Mesh Variation”.
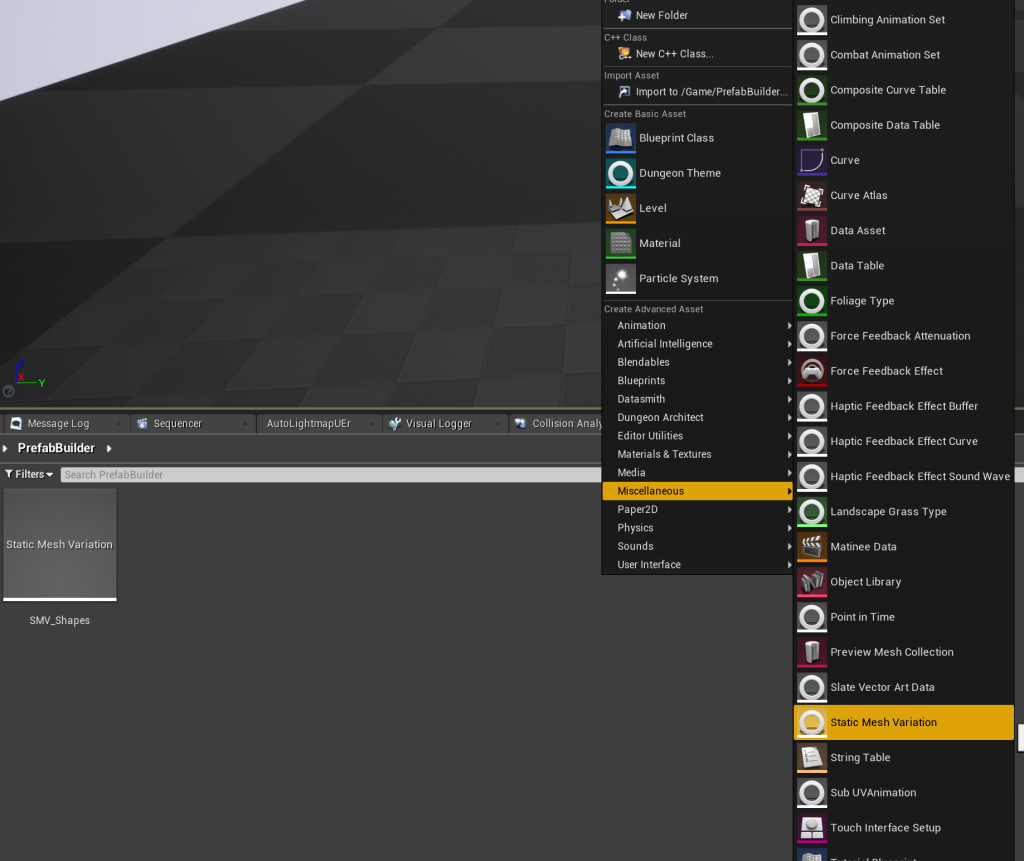